Just asked chatGPT to code in Angular, Angular Material, and Velo (Wix)
When artificial intelligence may finally take away repetitive and boring tasks!
On the last part of my book, I have interviewed chatGPT.
What about coding?
Heard a story using pure HTML and wanted to try out using a framework instead!
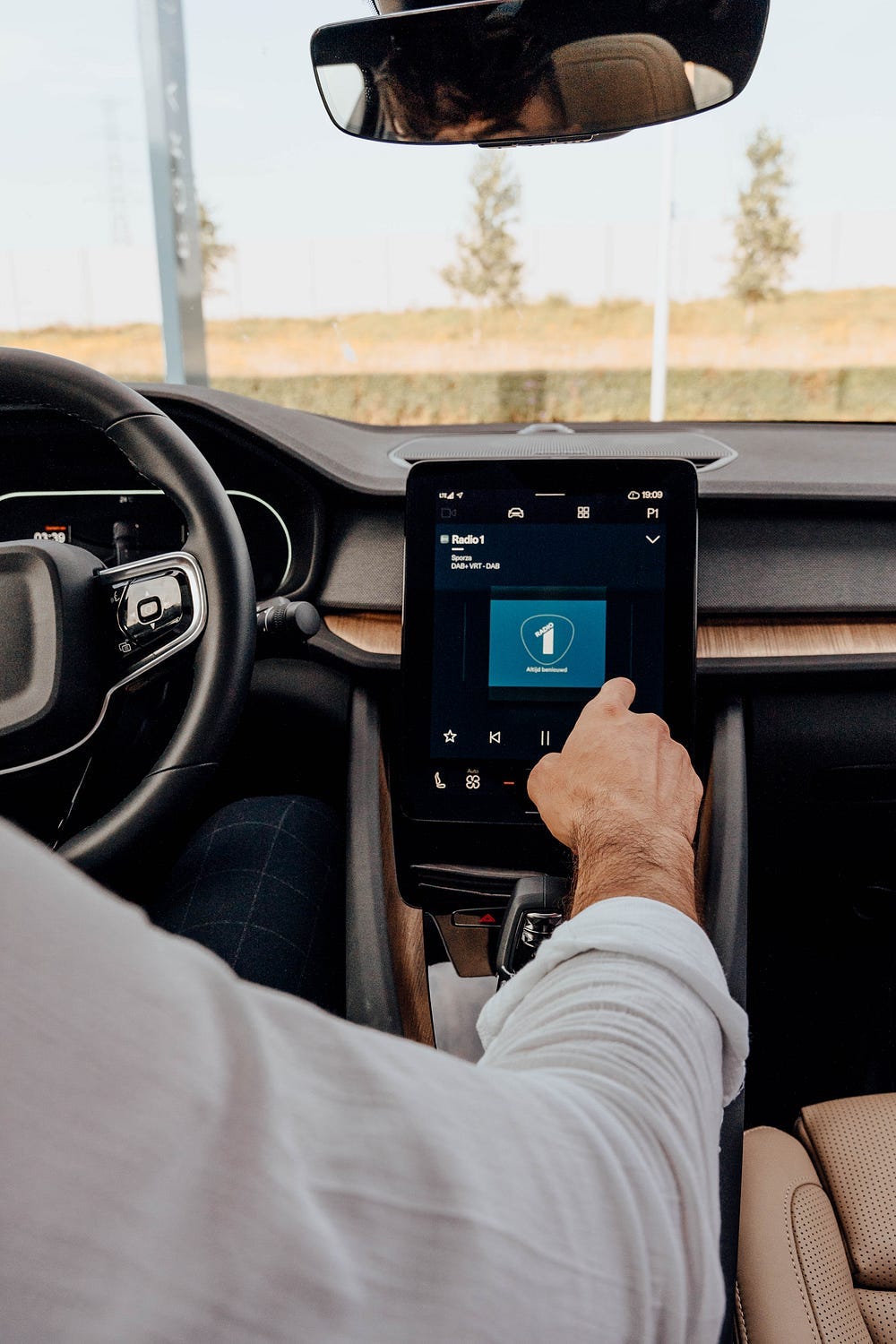
Create a button using Angular Material
Output:
Here is an example of how you can create a button using Angular Material:
<button mat-raised-button (click)="onClick()">Click me</button>
In this example, the mat-raised-button
directive is used to create a Material Design-styled button. The (click)
event binding is used to call the onClick()
method in the component's class when the button is clicked.
You need to import MatButtonModule
from the @angular/material
library in the app.module.ts
file and add it to the imports
array of the @NgModule
decorator.
import { BrowserModule } from '@angular/platform-browser';
import { NgModule } from '@angular/core';
import { MatButtonModule } from '@angular/material/button';
import { AppComponent } from './app.component';
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
MatButtonModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
You also need to add the following line to the styles array in your angular.json
file:
"node_modules/@angular/material/prebuilt-themes/indigo-pink.css"
And in the component class you can add the onClick()
method:Copy code
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
template: `
<button mat-raised-button (click)="onClick()">Click me</button>
`,
})
export class AppComponent {
onClick() {
console.log("Button clicked!");
}
}
The onClick()
method simply logs a message to the console when the button is clicked.
Here goes my favorite part:
Don’t forget to run
ng serve
in your command line to run the application in the browser.
Velo (Wix programming language)
This one is interesting Velo, a Javascript based language used to code in Wix, is not that well-known.
Using velo by wix, give me the code to access a single element from a collection on a database on a Wix website
Here is an example code snippet in Velo by Wix to access a single element from a collection on a database:
wixData.query("CollectionName")
.eq("fieldName", "fieldValue")
.find()
.then((results) => {
let firstResult = results.items[0];
// access data from the firstResult object as needed
})
.catch((error) => {
console.log(error);
});
The comment was not added by me, it was chatGPT commenting the code!
Replace “CollectionName” with the actual name of your collection, and “fieldName” and “fieldValue” with the appropriate field name and value to filter your results. This code uses the wixData.query()
method to query the collection, and the eq()
method to filter the results based on a specific field value. The find()
method is then used to retrieve the filtered results, and the then()
method is used to access the first result in the returned array of items.
Final remarks
Sounds great! Did not test bigger code block, but it is already awesome! I have seen on YouTube people creating even more complex codes, such as Tic Tac Toe! This code can take days to create even the simplest interface; not to mention that you may be tired, as happened to me when creating a machine learning based Tic Tac Toe!